I. Introduction
A. Overview Of Flutter And Its Package Ecosystem
Flutter is a popular open-source UI toolkit developed by Google for building natively compiled applications for mobile, web, and desktop platforms from a single codebase. It provides a rich set of pre-built widgets and tools that enable developers to create beautiful and performant apps.
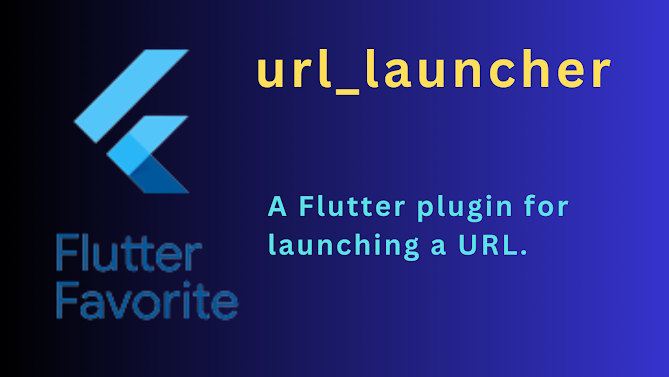
One of the key advantages of Flutter is its extensive package ecosystem, which offers a wide range of third-party libraries and packages that extend the functionality of Flutter apps. These packages cover various domains, including UI components, networking, database access, and more.
B. Importance Of Url Launching In Mobile Apps
URL launching plays a vital role in mobile apps as it allows developers to seamlessly integrate their applications with external resources, such as websites, other apps, or specific actions. By launching URLs, developers can enhance the user experience, provide deep linking capabilities, enable social sharing, and leverage various platform-specific features.
URL launching is particularly important in Flutter apps as it enables users to interact with external content, ensuring a smooth integration between the app and the broader mobile ecosystem. Whether it's opening a website, sharing content on social media, or initiating phone calls, URL launching empowers developers to build feature-rich and interconnected applications.
C. Introduction To The "Url_launcher" Package And Its Purpose
The "url_launcher" package is a widely adopted Flutter package that simplifies the process of launching URLs in Flutter apps. It provides a convenient API for opening URLs in the default browser or specific applications installed on the user's device. The package supports both Android and iOS platforms, making it a versatile solution for URL handling in Flutter projects.
Ii. Getting Started With "Url_launcher"
A. Installation And Setup Instructions
To begin using the "url_launcher" package, follow these simple installation steps:
1. Open your Flutter project in an editor of your choice.
2. Locate the `pubspec.yaml` file in the root directory of your project.
3. Add the following line to the `dependencies` section of the `pubspec.yaml` file:
```yaml
dependencies:
url_launcher: ^6.0.0
```
4. Save the `pubspec.yaml` file and run the following command in the terminal to fetch the package:
```bash
flutter pub get
```
B. Importing The Package Into A Flutter Project
After the package is successfully installed, you can import it into your Flutter project. Add the following line at the top of your Dart file:
```dart
import 'package:url_launcher/url_launcher.dart';
```
C. Compatibility And Platform Support
The "url_launcher" package is compatible with Flutter projects targeting Android and iOS platforms. It supports Flutter versions 1.20.0 and higher, ensuring compatibility with most Flutter projects.
Iii. Basic Usage
A. Launching A Url In The Default Browser
To launch a URL in the default browser, use the `launch` function provided by the "url_launcher" package. Here's an example:
```dart
void launchURL() async {
const url = 'https://example.com';
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
```
In this code snippet, we define a function `launchURL` that launches the URL `https://example.com` using the `launch` function. The `canLaunch` function is used to check if the device is capable of handling the URL before launching it.
B. Opening A Url In A Specific Browser
The "url_launcher" package also allows opening a URL in a specific browser installed on the device. Here's an example:
```dart
void launchURLInBrowser() async {
const url
= 'https://example.com';
if (await canLaunch(url)) {
await launch(url, forceSafariVC: false, universalLinksOnly: true);
} else {
throw 'Could not launch $url';
}
}
```
In this code snippet, we use the `launch` function with additional parameters `forceSafariVC` and `universalLinksOnly` to specify that we want to open the URL in the default browser rather than a Safari view controller on iOS.
C. Handling Unsupported Urls Gracefully
Sometimes, a device may not have a suitable app or browser to handle a specific URL scheme. To handle such scenarios gracefully, the "url_launcher" package provides the `canLaunch` function. Here's an example:
```dart
void checkURLSupport() async {
const unsupportedUrl = 'scheme://unsupported';
if (await canLaunch(unsupportedUrl)) {
await launch(unsupportedUrl);
} else {
print('The URL $unsupportedUrl is not supported on this device.');
}
}
```
In this code snippet, we use the `canLaunch` function to check if the device supports launching the specified URL. If it's unsupported, we display a message indicating that the URL is not supported on the device.
Iv. Advanced Usage
A. Launching Urls With Custom Headers
Sometimes, you may need to launch URLs with custom headers, such as authentication tokens or user agent strings. The "url_launcher" package allows you to pass custom headers using the `headers` parameter in the `launch` function. Here's an example:
```dart
void launchURLWithHeaders() async {
const url = 'https://example.com';
final headers = {'Authorization': 'Bearer token'};
if (await canLaunch(url)) {
await launch(url, headers: headers);
} else {
throw 'Could not launch $url';
}
}
```
In this code snippet, we pass a map of custom headers to the `launch` function using the `headers` parameter. This enables you to add headers such as authentication tokens or any other custom headers required by the server.
B. Deep Linking And Handling App-Specific Urls
Deep linking allows users to open a specific page or screen within an app directly from an external URL. The "url_launcher" package supports deep linking by launching app-specific URLs. Here's an example:
```dart
void launchAppSpecificURL() async {
const appURL = 'myapp://open/settings';
if (await canLaunch(appURL)) {
await launch(appURL);
} else {
throw 'Could not launch $appURL';
}
}
```
In this code snippet, we use the `launch` function to open the app-specific URL `myapp://open/settings`. If the app is installed on the device and supports the specified URL scheme, it will be launched with the corresponding screen or action.
C. Checking If A Url Can Be Launched
Before launching a URL, it's essential to check if the device supports handling it. The "url_launcher" package provides the `canLaunch` function to determine if a URL can be launched. Here's an example:
```dart
void checkURLLaunchability() async {
const url = 'https://example.com';
final isLaunchable = await canLaunch(url);
print('URL launchable: $isLaunchable');
}
```
In this code snippet, we use the `canLaunch` function to check if the URL `https://example.com` can be launched. The function returns a boolean value indicating whether the URL can be handled by the device.
V. Handling Errors And Edge Cases
A. Catching Exceptions And Handling Errors
When using the "url_launcher" package, it's important to handle exceptions and errors that may occur during URL launching. By using try-catch blocks, you can gracefully handle errors and provide appropriate feedback to the user. Here's an example:
```dart
void launchURLWithExceptionHandling() async {
const url = 'https://example.com';
try {
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
} catch (e) {
print('An error occurred: $e');
}
}
```
In this code snippet, we wrap the URL launching logic within a try-catch block to catch any exceptions that may occur. If an exception is caught, we display an error message containing the exception details.
B. Dealing With Unavailable Network Connections
In scenarios where the device has no network connection or the requested URL is not accessible, it's important to handle such cases gracefully. The "url_launcher" package does not provide direct network connectivity checks, but you can leverage other packages or platform-specific APIs to determine network availability.
```dart
import 'package:connectivity/connectivity.dart';
void launchURLWithNetworkCheck() async {
const url = 'https://example.com';
final connectivityResult = await Connectivity().checkConnectivity();
if (connectivityResult != ConnectivityResult.none) {
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
} else {
print('No network connection available.');
}
}
```
In this code snippet, we use the `connectivity` package to check the device's network connectivity using the `checkConnectivity` method. If the device has an active network connection, the URL is launched; otherwise, a message is displayed indicating the unavailability of a network connection.
C. Handling Scenarios Where No Browsers Are Available
In some cases, a device may not have any browsers installed or available to handle the launched URL. To handle such scenarios, you can provide a fallback option or alternative behavior to the user. Here's an example:
```dart
void launchURLWithFallback() async {
const url = 'https://example.com';
if (await canLaunch(url)) {
await launch(url);
} else {
// Fallback behavior, such as opening the URL in a WebView within the app.
print('No browsers available to handle the URL.');
}
}
```
In this code snippet, if the device doesn't have any browsers capable of handling the URL, we display a message indicating the unavailability of browsers. You can also provide alternative behavior, such as opening the URL within a WebView embedded within the app.
Vi. Customization And Ui Integration
A. Creating Custom Buttons Or Widgets For Url Launching
To provide a seamless user experience, you can create custom buttons or widgets in your Flutter app for launching URLs. By combining the "url_launcher" package with Flutter's UI capabilities, you can design visually appealing components that trigger URL launches when interacted with.
Here's an example of a custom button widget that launches a URL:
```dart
import 'package:flutter/material.dart';
class URLLaunchButton extends StatelessWidget {
final String url;
final String buttonText;
const URLLaunchButton({required this.url, required this.buttonText});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: () => launchURL(),
child: Text(buttonText),
);
}
Future<void> launchURL() async {
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
}
```
In this code snippet, we create a custom button widget `URLLaunchButton` that takes a `url` and `buttonText` as parameters. The button triggers the `launchURL` method when pressed, which launches the specified URL using the "url_launcher" package.
B. Designing In-App Web Views For A Seamless User Experience
In addition to launching URLs in external browsers, you may also want to provide an in-app web browsing experience for certain URLs. Flutter provides the "webview_flutter" package, which can be integrated with the "url_launcher" package to display web content within your app.
To use the "webview_flutter" package, you need to add it to your `pubspec.yaml` file and import it into your Dart file. You can then create a WebView widget and load the desired URL. Here's a simple example:
```dart
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
class WebViewScreen extends StatelessWidget {
final String url;
const WebViewScreen({required this.url});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('In-App Web View'),
),
body: WebView(
initialUrl: url,
),
);
}
}
```
In this code snippet, we create a `WebViewScreen` widget that displays an in-app web view using the "webview_flutter" package. The widget takes a `url` parameter and renders a Scaffold with an AppBar and a WebView widget, which loads the specified URL.
C. Enhancing Ui With Progress Indicators And Error Messages
When launching URLs or loading web content, it's important to provide visual feedback to the user. You can enhance the user interface by adding progress indicators or error messages to indicate the loading state or any potential errors that may occur.
Here's an example of a modified `WebViewScreen` widget that includes a progress indicator:
```dart
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
class WebViewScreen extends StatefulWidget {
final String url;
const WebViewScreen({required this.url});
@override
_WebViewScreenState createState() => _WebViewScreenState();
}
class _WebViewScreenState extends State<WebViewScreen> {
bool _isLoading = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('In-App Web View'),
),
body: Stack(
children: [
WebView(
initialUrl: widget.url,
onPageStarted: (url) => setState(() => _isLoading = true),
onPageFinished: (url) => setState(() => _isLoading = false),
),
if (_isLoading)
Center(
child: CircularProgressIndicator(),
),
],
),
);
}
}
```
In this code snippet, we introduce a `_isLoading` state variable to track the loading state of the web view. We use a `Stack` widget to overlay a `CircularProgressIndicator` at the center of the screen while the content is loading. The `_isLoading` state is updated using the `onPageStarted` and `onPageFinished` callbacks of the WebView widget.
Vii. Best Practices And Tips
A. Avoiding Common Pitfalls And Mistakes
When working with the "url_launcher" package, it's important to follow best practices to ensure a smooth and error-free user experience. Here are some tips to avoid common pitfalls:
- Always check if a URL can be launched using the `canLaunch` function before calling `launch` to prevent crashes or exceptions.
- Handle exceptions and errors that may occur during URL launching to provide appropriate feedback to the user.
- Gracefully handle
scenarios where no browsers or suitable apps are available to handle the launched URLs.
- Test your app on different devices and platforms to ensure compatibility and consistent behavior.
B. Managing Url Schemes And Deep Linking Efficiently
When working with deep linking and app-specific URLs, it's crucial to manage URL schemes efficiently. Here are some tips:
- Define and document the URL schemes and deeplink patterns used by your app.
- Handle deep links and app-specific URLs by checking the URL scheme and path segments.
- Use platform-specific code or plugins to handle deeplinks and app-specific URL launches.
- Test deep linking functionality thoroughly on both Android and iOS devices.
C. Optimizing Performance And Resource Usage
To optimize performance and resource usage when working with the "url_launcher" package, consider the following tips:
- Avoid launching URLs unnecessarily. Only trigger URL launches when necessary to minimize the number of context switches and improve app performance.
- Cache and reuse instances of the `canLaunch` function to avoid repetitive checks for the same URL.
- Handle network connectivity gracefully and provide appropriate feedback to the user when URLs cannot be launched due to unavailability of a network connection.
Viii. Real-World Examples And Use Cases
A. Implementing Social Media Sharing In An App
The "url_launcher" package is often used to implement social media sharing functionality in Flutter apps. For example, you can use the package to launch URLs that prepopulate social media post content. Here's an example of launching a Twitter sharing URL:
```dart
void shareOnTwitter() async {
const url = 'https://twitter.com/intent/tweet?text=Hello%20world!';
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
```
In this code snippet, we launch a Twitter sharing URL that prepopulates the tweet with the text "Hello world!". This enables users to share content from your app directly to Twitter.
B. Opening Email Or Phone Number Links With Appropriate Apps
The "url_launcher" package is also useful for opening email or phone number links with the appropriate apps on the device. Here's an example of launching an email app with a pre-filled recipient and subject:
```dart
void sendEmail() async {
const url = 'mailto:recipient@example.com?subject=Hello%20from%20Flutter!';
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
```
In this code snippet, we launch an email app with the recipient and subject pre-filled. This allows users to compose an email with the provided details directly from your app.
C. Integrating With Maps And Navigation Services
The "url_launcher" package can be used to integrate with maps and navigation services. For example, you can launch map URLs to display locations or initiate navigation. Here's an example of launching a Google Maps URL with a specified location:
```dart
void openLocationInMaps() async {
const url = 'https://www.google.com/maps/search/?api=1&query=latitude,longitude';
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
```
In this code snippet, we launch a Google Maps URL that searches for a location based on latitude and longitude coordinates. This allows users to view the location in the Google Maps app or initiate navigation to that location.
Ix. Conclusion
In conclusion, the "url_launcher" package is a powerful tool for launching URLs in Flutter apps, providing seamless integration with the device's browsers and apps. In this article, we explored the various features and capabilities of the package, including basic and advanced usage, error handling, customization, and best practices. We also discussed real-world examples and use cases where the "url_launcher" package can be leveraged to enhance user experiences and enable integration with external services. By following the guidelines and examples provided, you can easily incorporate URL launching functionality into your Flutter apps and provide a seamless browsing and navigation experience for your users. We encourage you to explore and experiment with the "url_launcher" package to unlock its full potential in your projects.
0 Comments
Welcome! Please Comment Without Any Hesitation.
Thank You